WordPress カスタム投稿でCSV一括ダウンロードする
前回の記事「カスタム投稿でCSV一括アップロードする(複数カスタムフィールド対応)」で、複数のカスタムフィールドを持つカスタム投稿タイプに対してCSVで一括アップロードする方法を書きました。
CSVデータをアップロードしてカスタム投稿タイプに一括で投稿する事ができましたが、次に投稿を更新するために登録された投稿のIDを元のCSVデータに入力する必要が出てきます。
投稿数が少なければ手動でできそうですが、投稿が数百件にもなると骨が折れてしまいそうな作業ですので、カスタム投稿のアップロードとは逆のダウンロードについて実装していきます。
アップロードにはWordPressプラグイン「Really Simple CSV Importer」を利用しましたが、ダウンロードはプラグインなしでfunctions.phpなどに直接書く方法で実装します。
カスタム投稿の一覧画面にダウンロードボタンを追加する
カスタム投稿の一覧ページを開くと以下のような画面となりますが、この絞り込みの右側にCSVダウンロードボタンを追加します。
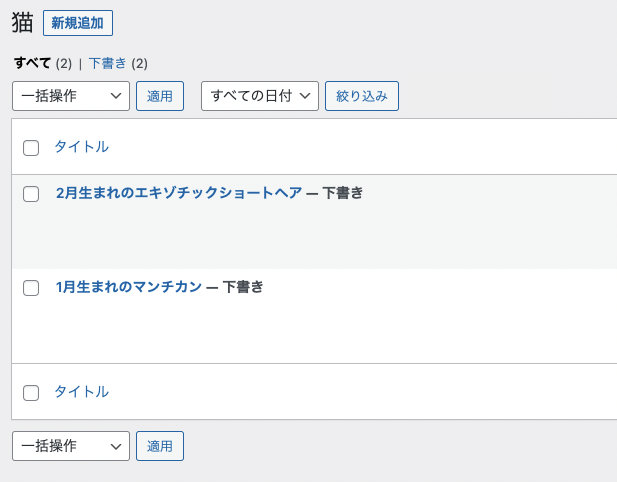
CSVダウンロードボタンを追加するソースコードは以下となります。
if ( !function_exists( 'cat_manage_posts_extra_tablenav' ) ) {
function cat_manage_posts_extra_tablenav($which) {
global $typenow;
if ( $typenow === 'cat' && $which === 'top' ) {
wp_nonce_field('posttype-cat-csv-download-nonce-action', 'posttype-cat-csv-download-nonce-key');
echo '<input type="submit" name="export_all_posts" class="button" value="CSV ダウンロード" />';
}
}
add_action( 'manage_posts_extra_tablenav', 'cat_manage_posts_extra_tablenav' , 10, 2 );
}
アクションフック「manage_posts_extra_tablenav」を使う事で絞り込みの右側にHTML要素を追加する事ができます。
$typenowで現在の投稿タイプ=カスタム投稿タイプ「猫」の判定を行い、合わせて $which === ‘top’ で投稿一覧の上部かどうかを判定しています($which === ‘bottom’ とする事で投稿一覧の下部に追加する事も可能です)。
上記ソースコードを適用してエラーなく問題ない場合は以下のようにCSVダウンロードボタンが追加されます。
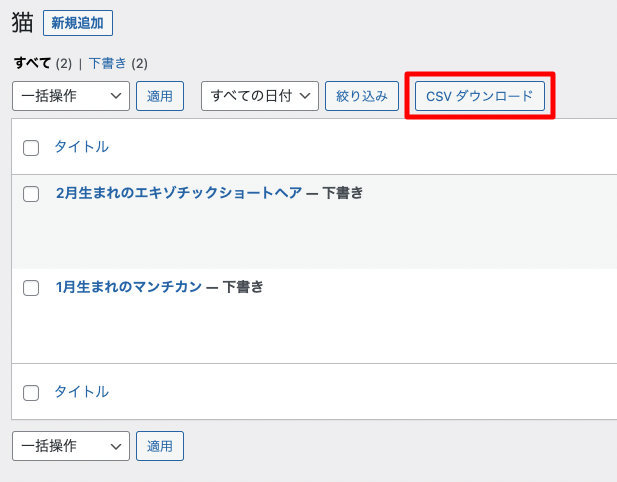
上記のソースコードはCSVダウンロードボタンの追加のみとなりますので、CSVダウンロードが押された時の動作を実装していきます。
カスタム投稿一覧のCSVダウンロードの実装
カスタム投稿一覧をCSVファイルとしてダウンロードするためのソースコードを以下に記載します。
if ( !function_exists( 'posttype_cat_csv_download' ) ) {
function posttype_cat_csv_download() {
if (isset($_GET['posttype-cat-csv-download-nonce-key']) && $_GET['posttype-cat-csv-download-nonce-key']) :
if (check_admin_referer('posttype-cat-csv-download-nonce-action', 'posttype-cat-csv-download-nonce-key')) :
if ( isset( $_GET['export_all_posts'] ) ) :
$csv = array();
$args = array(
'post_type' => 'cat',
'posts_per_page' => -1,
);
$query = new WP_Query($args);
if($query -> have_posts()):
$csv = array(
0 => array(
'post_id',
'post_name',
'post_author',
'post_date',
'post_type',
'post_status',
'post_title',
'cat_age',
'cat_sex',
'tax_cat_genre',
),
);
while($query -> have_posts()): $query -> the_post();
$terms = get_the_terms(get_the_ID(), 'cat_genre');
$terms_array = array();
if ( $terms ) :
foreach ( $terms as $term ) {
array_push($terms_array, $term->name);
}
endif;
$result_terms = implode( ',', $terms_array );
$cat_age = get_post_meta( get_the_ID(), 'cat_age', true );
$cat_sex = get_post_meta( get_the_ID(), 'cat_sex', true );
$csv += array(
get_the_ID() => array(
get_the_ID(),
get_post( get_the_ID() )->post_name,
get_the_author(),
get_the_date(),
get_post_type(),
get_post_status(),
get_the_title(),
$cat_age,
$cat_sex,
$result_terms,
),
);
endwhile;
endif;
wp_reset_postdata();
try {
$csvFileName = dirname(__FILE__).'/posttype_cat_download.csv';
$res = fopen($csvFileName, 'w');
if ($res === FALSE) {
throw new Exception('ファイルの書き込みに失敗しました。');
}
foreach($csv as $k => $v) {
fputcsv($res, $v);
}
fclose($res);
header('Content-Type: application/octet-stream');
header('Content-Disposition: attachment; filename="posttype_cat_download.csv"');
header('Pragma: no-cache');
header('Expires: 0');
header('Content-Length: ' . filesize($csvFileName));
readfile($csvFileName);
unlink($csvFileName);
exit;
} catch(Exception $e) {
// echo $e->getMessage();
}
endif;
endif;
endif;
}
add_action( 'init', 'posttype_cat_csv_download' );
}
アクションフック「manage_posts_extra_tablenav」で使いされたボタンのname属性値 export_all_posts はGETパラメータで受け取る事ができます。
nonceが生成されているかの確認とissetでGETパラメータの存在確認を行い、その後 $csv 配列に保存するフィールド名(カスタムフィールド含む)を代入していきます。
最終的な配列 $csv の組み立てが完了しましたら、try ~ catch 箇所で CSVのダウンロード処理を行います。
上記のソースコードで問題なく実行ができた場合、posttype_cat_download.csv というCSVファイルがダウンロードできます。

ICTよろず相談所ではWordPressのカスタム投稿・プラグインの導入・カスタマイズなどのご相談も承っております。WordPressに関するご依頼はお問い合わせよりご連絡ください。